Work with Roles using System; using Microsoft.SharePoint.Client; namespace Microsoft.SDK.SharePointServices.Samples { class CreateRoleDefinition { static void Main() { ClientContext oClientContext = new ClientContext("http://MyServer/sites/MySiteCollection"); Web oWebsite = clientContext.Web; BasePermissions permissions = new BasePermissions(); permissions.Set(PermissionKind.CreateAlerts); permissions.Set(PermissionKind.ManageAlerts); RoleDefinitionCreationInformation roleCreationInfo = new RoleDefinitionCreationInformation(); roleCreationInfo.BasePermissions = permissions; roleCreationInfo.Description = "A new role with create and manage alerts permission"; roleCreationInfo.Name = "Create and Manage Alerts"; roleCreationInfo.Order = 4; RoleDefinition oRoleDefinition = oWebsite.RoleDefinitions.Add(roleCreationInfo); clientContext.ExecuteQuery(); Console.WriteLine("{0} role created.", oRoleDefinition.Name); } } } Retrieving all users from a SharePoint group using System; using Microsoft.SharePoint.Client; namespace Microsoft.SDK.SharePointServices.Samples { class RetrieveAllUsersInGroup { static void Main() { ClientContext clientContext = new ClientContext("http://MyServer/sites/MySiteCollection"); GroupCollection collGroup = clientContext.Web.SiteGroups; Group oGroup = collGroup.GetById(7); UserCollection collUser = oGroup.Users; clientContext.Load(collUser); clientContext.ExecuteQuery(); foreach (User oUser in collUser) { Console.WriteLine("User: {0} ID: {1} Email: {2} Login Name: {3}", oUser.Title, oUser.Id, oUser.Email, oUser.LoginName); } } } } Create, Update, and Delete List Items using System; using Microsoft.SharePoint.Client; using SP = Microsoft.SharePoint.Client; namespace Microsoft.SDK.SharePointServices.Samples { class CreateListItem { static void Main() { string siteUrl = "http://MyServer/sites/MySiteCollection"; ClientContext clientContext = new ClientContext(siteUrl); SP.List oList = clientContext.Web.Lists.GetByTitle("Announcements"); ListItemCreationInformation itemCreateInfo = new ListItemCreationInformation(); ListItem oListItem = oList.AddItem(itemCreateInfo); oListItem["Title"] = "My New Item!"; oListItem["Body"] = "Hello World!"; oListItem.Update(); clientContext.ExecuteQuery(); } } } Updating a list item using System; using Microsoft.SharePoint.Client; using SP = Microsoft.SharePoint.Client; namespace Microsoft.SDK.SharePointServices.Samples { class UpdateListItem { static void Main() { string siteUrl = "http://MyServer/sites/MySiteCollection"; ClientContext clientContext = new ClientContext(siteUrl); SP.List oList = clientContext.Web.Lists.GetByTitle("Announcements"); ListItem oListItem = oList.Items.GetById(3); oListItem["Title"] = "My Updated Title."; oListItem.Update(); clientContext.ExecuteQuery(); } } } Deleting a list item using System; using Microsoft.SharePoint.Client; using SP = Microsoft.SharePoint.Client; namespace Microsoft.SDK.SharePointServices.Samples { class DeleteListItem { static void Main() { string siteUrl = "http://MyServer/sites/MySiteCollection"; ClientContext clientContext = new ClientContext(siteUrl); SP.List oList = clientContext.Web.Lists.GetByTitle("Announcements"); ListItem oListItem = oList.GetItemById(2); oListItem.DeleteObject(); clientContext.ExecuteQuery(); } } } ---------Or------ using System; using Microsoft.SharePoint.Client; using SP = Microsoft.SharePoint.Client; namespace Microsoft.SDK.SharePointServices.Samples { class DeleteListItemDisplayCount { static void Main() { string siteUrl = "http://MyServer/sites/MySiteCollection"; ClientContext clientContext = new ClientContext(siteUrl); SP.List oList = clientContext.Web.Lists.GetByTitle("Announcements"); clientContext.Load(oList, list => list.ItemCount); clientContext.ExecuteQuery(); int startCount = oList.ItemCount; ListItem oListItem = oList.GetItemById(2); oListItem.DeleteObject(); oList.Update(); clientContext.Load(oList, list => list.ItemCount); clientContext.ExecuteQuery(); int endCount = oList.ItemCount; Console.WriteLine("Start: {0} End: {1}", startCount, endCount); } } } Retrieve List Items using System; using Microsoft.SharePoint.Client; using SP = Microsoft.SharePoint.Client; namespace Microsoft.SDK.SharePointServices.Samples { class RetrieveListItems { static void Main() { string siteUrl = "http://MyServer/sites/MySiteCollection"; ClientContext clientContext = new ClientContext(siteUrl); SP.List oList = clientContext.Web.Lists.GetByTitle("Announcements"); CamlQuery camlQuery = new CamlQuery(); camlQuery.ViewXml = "<View><Query><Where><Geq><FieldRef Name='ID'/>" + "<Value Type='Number'>10</Value></Geq></Where></Query><RowLimit>100</RowLimit></View>"; ListItemCollection collListItem = oList.GetItems(camlQuery); clientContext.Load(collListItem); clientContext.ExecuteQuery(); foreach (ListItem oListItem in collListItem) { Console.WriteLine("ID: {0} \nTitle: {1} \nBody: {2}", oListItem.Id, oListItem["Title"], oListItem["Body"]); } } } } Using the Include method using System; using System.Linq; using Microsoft.SharePoint.Client; using SP = Microsoft.SharePoint.Client; namespace Microsoft.SDK.SharePointServices.Samples { class RetrieveListItemsInclude { static void Main() { string siteUrl = "http://MyServer/sites/MySiteCollection"; ClientContext clientContext = new ClientContext(siteUrl); SP.List oList = clientContext.Web.Lists.GetByTitle("Announcements"); CamlQuery camlQuery = new CamlQuery(); camlQuery.ViewXml = "<View><RowLimit>100</RowLimit></View>"; ListItemCollection collListItem = oList.GetItems(camlQuery); clientContext.Load(collListItem, items => items.Include( item => item.Id, item => item.DisplayName, item => item.HasUniqueRoleAssignments)); clientContext.ExecuteQuery(); foreach (ListItem oListItem in collListItem) { Console.WriteLine("ID: {0} \nDisplay name: {1} \nUnique role assignments: {2}", oListItem.Id, oListItem.DisplayName, oListItem.HasUniqueRoleAssignments); } } } } Retrieving specific fields from a specified number of items using System; using System.Linq; using Microsoft.SharePoint.Client; using SP = Microsoft.SharePoint.Client; namespace Microsoft.SDK.SharePointServices.Samples { class RetrieveSpecificItemsFields { static void Main() { string siteUrl = "http://MyServer/sites/MySiteCollection"; ClientContext clientContext = new ClientContext(siteUrl); SP.List oList = clientContext.Web.Lists.GetByTitle("Announcements"); CamlQuery camlQuery = new CamlQuery(); ListItemCollection collListItem = oList.GetItems(camlQuery); clientContext.Load( collListItem, items => items.Take(5).Include( item => item["Title"], item => item["Body"])); clientContext.ExecuteQuery(); foreach (ListItem oListItem in collListItem) { Console.WriteLine("Title: {0} \nBody: {1}\n", oListItem["Title"], oListItem["Body"]); } } } } Retrieving items from all the lists in a Web site using System; using System.Linq; using Microsoft.SharePoint.Client; using SP = Microsoft.SharePoint.Client; namespace Microsoft.SDK.SharePointServices.Samples { class RetrieveFirstTenItemsAllLists { static void Main() { string siteUrl = "http://MyServer/sites/MySiteCollection"; ClientContext clientContext = new ClientContext(siteUrl); ListCollection collList = clientContext.Web.Lists; clientContext.Load( collList, lists => lists.Where( list => list.Hidden == false).Include( list => list.Title, list => list.Items.Take(10))); clientContext.ExecuteQuery(); foreach (SP.List oList in clientContext.Web.Lists) { string listTitle = oList.Title; int itemCount = oList.Items.Count; Console.WriteLine("List {0} returned with {1} items", listTitle, itemCount); } } } } Retrieving items using list item collection position using System; using System.Linq; using Microsoft.SharePoint.Client; using SP = Microsoft.SharePoint.Client; namespace Microsoft.SDK.SharePointServices.Samples { class UsingItemCollectionPosition { static void Main() { string siteUrl = "http://MyServer/sites/MySiteCollection"; ClientContext clientContext = new ClientContext(siteUrl); SP.List oList = clientContext.Web.Lists.GetByTitle("Announcements"); ListItemCollectionPosition itemPosition = null; while (true) { CamlQuery camlQuery = new CamlQuery(); camlQuery.ListItemCollectionPosition = itemPosition; camlQuery.ViewXml = "<View><ViewFields><FieldRef Name='ID'/>" + "<FieldRef Name='Title'/><FieldRef Name='Body'/>" + "</ViewFields><RowLimit>5</RowLimit></View>"; ListItemCollection collListItem = oList.GetItems(camlQuery); clientContext.Load(collListItem); clientContext.ExecuteQuery(); itemPosition = collListItem.ListItemCollectionPosition; foreach (ListItem oListItem in collListItem) { Console.WriteLine("Title: {0}: \nBody: {1}", oListItem["Title"], oListItem["Body"]); } if (itemPosition == null) { break; } Console.WriteLine("\n" + itemPosition.PagingInfo + "\n"); } } } } Create, Update, and Delete Lists using System; using Microsoft.SharePoint.Client; namespace Microsoft.SDK.SharePointServices.Samples { class CreateList { static void Main() { string siteUrl = "http://MyServer/sites/MySiteCollection"; ClientContext clientContext = new ClientContext(siteUrl); Web oWebsite = clientContext.Web; ListCreationInformation listCreationInfo = new ListCreationInformation(); listCreationInfo.Title = "My Announcements List"; listCreationInfo.TemplateType = (int)ListTemplateType.Announcements; List oList = oWebsite.Lists.Add(listCreationInfo); clientContext.ExecuteQuery(); } } } Adding a field to a list using System; using Microsoft.SharePoint.Client; using SP = Microsoft.SharePoint.Client; namespace Microsoft.SDK.SharePointServices.Samples { class Program { static void Main() { string siteUrl = "http://MyServer/sites/MySiteCollection"; ClientContext clientContext = new ClientContext(siteUrl); SP.List oList = clientContext.Web.Lists.GetByTitle("Announcements"); SP.Field oField = oList.Fields.AddFieldAsXml("<Field DisplayName='MyField' Type='Number' />", true, AddFieldOptions.DefaultValue); SP.FieldNumber fieldNumber = clientContext.CastTo<FieldNumber>(oField); fieldNumber.MaximumValue = 100; fieldNumber.MinimumValue = 35; fieldNumber.Update(); clientContext.ExecuteQuery(); } } } Deleting a list using System; using Microsoft.SharePoint.Client; namespace Microsoft.SDK.SharePointServices.Samples { class DeleteList { static void Main() { string siteUrl = "http://MyServer/sites/MySiteCollection"; ClientContext clientContext = new ClientContext(siteUrl); Web oWebsite = clientContext.Web; List oList = oWebsite.Lists.GetByTitle("My Announcements List"); oList.DeleteObject(); clientContext.ExecuteQuery(); } } } Retrieving all properties of all lists in a Web site using System; using Microsoft.SharePoint.Client; using SP = Microsoft.SharePoint.Client; namespace Microsoft.SDK.SharePointServices.Samples { class RetrieveAllListProperties { static void Main() { string siteUrl = "http://MyServer/sites/MySiteCollection"; ClientContext clientContext = new ClientContext(siteUrl); Web oWebsite = clientContext.Web; ListCollection collList = oWebsite.Lists; clientContext.Load(collList); clientContext.ExecuteQuery(); foreach (SP.List oList in collList) { Console.WriteLine("Title: {0} Created: {1}", oList.Title, oList.Created.ToString()); } } } } Retrieving only specified properties of lists using System; using Microsoft.SharePoint.Client; using SP = Microsoft.SharePoint.Client; namespace Microsoft.SDK.SharePointServices.Samples { class RetrieveSpecificListProperties { static void Main() { string siteUrl = "http://MyServer/sites/MySiteCollection"; ClientContext clientContext = new ClientContext(siteUrl); Web oWebsite = clientContext.Web; ListCollection collList = oWebsite.Lists; clientContext.Load( collList, lists => lists.Include( list => list.Title, list => list.Id)); clientContext.ExecuteQuery(); foreach (SP.List oList in collList) { Console.WriteLine("Title: {0} ID: {1}", oList.Title, oList.Id.ToString("D")); } } } } Storing retrieved lists in a collection using System; using System.Collections.Generic; using Microsoft.SharePoint.Client; using SP = Microsoft.SharePoint.Client; namespace Microsoft.SDK.SharePointServices.Samples { class RetrieveSpecificListPropertiesToCollection { static void Main() { string siteUrl = "http://MyServer/sites/MySiteCollection"; ClientContext clientContext = new ClientContext(siteUrl); Web oWebsite = clientContext.Web; ListCollection collList = oWebsite.Lists; IEnumerable<SP.List> resultCollection = clientContext.LoadQuery( collList.Include( list=>list.Title, list=>list.Id)); clientContext.ExecuteQuery(); foreach (SP.List oList in resultCollection) { Console.WriteLine("Title: {0} ID: {1}", oList.Title, oList.Id.ToString("D")); } } } } Retrieving list fields from a Web site using System; using System.Collections.Generic; using System.Text.RegularExpressions; using Microsoft.SharePoint.Client; using SP = Microsoft.SharePoint.Client; namespace Microsoft.SDK.SharePointServices.Samples { class RetrieveSpecificListPropertiesToCollection { static void Main() { string siteUrl = "http://MyServer/sites/MySiteCollection"; ClientContext clientContext = new ClientContext(siteUrl); Web oWebsite = clientContext.Web; ListCollection collList = oWebsite.Lists; IEnumerable<SP.List> listInfo = clientContext.LoadQuery( collList.Include( list => list.Title, list => list.Fields.Include( field => field.Title, field => field.InternalName))); clientContext.ExecuteQuery(); foreach (SP.List oList in listInfo) { FieldCollection collField = oList.Fields; foreach (SP.Field oField in collField) { Regex regEx = new Regex("name", RegexOptions.IgnoreCase); if (regEx.IsMatch(oField.InternalName)) { Console.WriteLine("List: {0} \n\t Field Title: {1} \n\t Field Internal Name: {2}", oList.Title, oField.Title, oField.InternalName); } } } } } } Use Conditional Scope using System; using System.Collections.Generic; using System.Linq; using System.Text; using Microsoft.SharePoint.Client; namespace Microsoft.SDK.SharePointServices.Samples { class Program { static void Main(string[] args) { ClientContext clientContext = new ClientContext("http://MyServer/sites/MySiteCollection"); Web oWebsite = clientContext.Web; List oList = oWebsite.GetCatalog((int)ListTemplateType.WebPartCatalog); BasePermissions permissions = new BasePermissions(); permissions.Set(PermissionKind.ManageLists); ConditionalScope scope = new ConditionalScope( clientContext, () => oList.ServerObjectIsNull.Value != true && oWebsite.DoesUserHavePermissions(permissions).Value == true); using (scope.StartScope()) { clientContext.Load(oList, list=>list.Title); } clientContext.ExecuteQuery(); Console.WriteLine(scope.TestResult.Value); if (scope.TestResult.Value) { Console.WriteLine(oList.Title); } } } } Work with Web Parts on a Page using System; using Microsoft.SharePoint.Client; using Microsoft.SharePoint.Client.WebParts; namespace SampleCode { class UpdateWebPartTitle { static void Main() { ClientContext oClientContext = new ClientContext("http://MyServer/sites/MySiteCollection"); File oFile = oClientContext.Web.GetFileByServerRelativeUrl("Default.aspx"); LimitedWebPartManager limitedWebPartManager = oFile.GetLimitedWebPartManager(PersonalizationScope.Shared); oClientContext.Load(limitedWebPartManager.WebParts, wps => wps.Include( wp => wp.WebPart.Title)); oClientContext.ExecuteQuery(); if (limitedWebPartManager.WebParts.Count == 0) { throw new Exception("No Web Parts on this page."); } WebPartDefinition oWebPartDefinition = limitedWebPartManager.WebParts[1]; WebPart oWebPart = oWebPartDefinition.WebPart; oWebPart.Title = "My New Web Part Title"; oWebPartDefinition.SaveWebPartChanges(); oClientContext.ExecuteQuery(); } } } Adding a Web Part to a page using System; using Microsoft.SharePoint.Client; using Microsoft.SharePoint.Client.WebParts; namespace SampleCode { class AddWebPart { static void Main() { ClientContext oClientContext = new ClientContext("http://MyServer/sites/MySiteCollection"); File oFile = oClientContext.Web.GetFileByServerRelativeUrl("Default.aspx"); LimitedWebPartManager limitedWebPartManager = oFile.GetLimitedWebPartManager(PersonalizationScope.Shared); string xmlWebPart = "<?xml version=\"1.0\" encoding=\"utf-8\"?>" + "<WebPart xmlns:xsi=\"http://www.w3.org/2001/XMLSchema-instance\"" + " xmlns:xsd=\"http://www.w3.org/2001/XMLSchema\"" + " xmlns=\"http://schemas.microsoft.com/WebPart/v2\">" + "<Title>My Web Part</Title><FrameType>Default</FrameType>" + "<Description>Use for formatted text, tables, and images.</Description>" + "<IsIncluded>true</IsIncluded><ZoneID></ZoneID><PartOrder>0</PartOrder>" + "<FrameState>Normal</FrameState><Height /><Width /><AllowRemove>true</AllowRemove>" + "<AllowZoneChange>true</AllowZoneChange><AllowMinimize>true</AllowMinimize>" + "<AllowConnect>true</AllowConnect><AllowEdit>true</AllowEdit>" + "<AllowHide>true</AllowHide><IsVisible>true</IsVisible><DetailLink /><HelpLink />" + "<HelpMode>Modeless</HelpMode><Dir>Default</Dir><PartImageSmall />" + "<MissingAssembly>Cannot import this Web Part.</MissingAssembly>" + "<PartImageLarge>/_layouts/images/mscontl.gif</PartImageLarge><IsIncludedFilter />" + "<Assembly>Microsoft.SharePoint, Version=13.0.0.0, Culture=neutral, " + "PublicKeyToken=94de0004b6e3fcc5</Assembly>" + "<TypeName>Microsoft.SharePoint.WebPartPages.ContentEditorWebPart</TypeName>" + "<ContentLink xmlns=\"http://schemas.microsoft.com/WebPart/v2/ContentEditor\" />" + "<Content xmlns=\"http://schemas.microsoft.com/WebPart/v2/ContentEditor\">" + "<![CDATA[This is a first paragraph!<DIV> </DIV>And this is a second paragraph.]]></Content>" + "<PartStorage xmlns=\"http://schemas.microsoft.com/WebPart/v2/ContentEditor\" /></WebPart>"; WebPartDefinition oWebPartDefinition = limitedWebPartManager.ImportWebPart(xmlWebPart); limitedWebPartManager.AddWebPart(oWebPartDefinition.WebPart, "Left", 1); oClientContext.ExecuteQuery(); } } } Deleting a Web Part from a page using System; using Microsoft.SharePoint.Client; using Microsoft.SharePoint.Client.WebParts; namespace SampleCode { class DeleteWebPart { static void Main() { ClientContext oClientContext = new ClientContext("http://MyServer/sites/MySiteCollection"); File oFile = oClientContext.Web.GetFileByServerRelativeUrl("/sites/MySiteCollection/SitePages/Home.aspx "); LimitedWebPartManager limitedWebPartManager = oFile.GetLimitedWebPartManager(PersonalizationScope.Shared); oClientContext.Load(limitedWebPartManager.WebParts); oClientContext.ExecuteQuery(); if (limitedWebPartManager.WebParts.Count == 0) { throw new Exception("No Web Parts to delete."); } WebPartDefinition webPartDefinition = limitedWebPartManager.WebParts[0]; webPartDefinition.DeleteWebPart(); oClientContext.ExecuteQuery(); } } } Work with User Custom Actions using System; using Microsoft.SharePoint.Client; namespace Microsoft.SDK.SharePointServices.Samples { class CreateUserCustomActionList { static void Main() { string urlWebsite = "http://MyServer/sites/MySiteCollection"; ClientContext clientContext = new ClientContext(urlWebsite); Web oWebsite = clientContext.Web; List oList = oWebsite.Lists.GetByTitle("My List"); UserCustomActionCollection collUserCustomAction = oList.UserCustomActions; UserCustomAction oUserCustomAction = collUserCustomAction.Add(); oUserCustomAction.Location = "EditControlBlock"; oUserCustomAction.Sequence = 100; oUserCustomAction.Title = "My First User Custom Action"; oUserCustomAction.Url = urlWebsite + @"/_layouts/MyPage.aspx"; oUserCustomAction.Update(); clientContext.Load(oList, list => list.UserCustomActions); clientContext.ExecuteQuery(); } } } Modifying a user custom action using System; using Microsoft.SharePoint.Client; namespace Microsoft.SDK.SharePointServices.Samples { class ModifyUserCustomAction { static void Main() { string urlWebsite = "http://MyServer/sites/SiteCollection"; ClientContext clientContext = new ClientContext(urlWebsite); Web oWebsite = clientContext.Web; List oList = oWebsite.Lists.GetByTitle("My List"); UserCustomActionCollection collUserCustomAction = oList.UserCustomActions; clientContext.Load(collUserCustomAction, userCustomActions => userCustomActions.Include( userCustomAction => userCustomAction.Title)); clientContext.ExecuteQuery(); foreach (UserCustomAction oUserCustomAction in collUserCustomAction) { if (oUserCustomAction.Title == "My First User Custom Action") { oUserCustomAction.ImageUrl = "http://MyServer/_layouts/images/MyIcon.png"; oUserCustomAction.Update(); clientContext.ExecuteQuery(); } } } } } Adding a user custom action to the site actions of a Web site using System; using Microsoft.SharePoint.Client; namespace Microsoft.SDK.SharePointServices.Samples { class CreateUserCustomActionSite { static void Main() { string urlWebsite = "http://MyServer/sites/MySiteCollection"; ClientContext clientContext = new ClientContext(urlWebsite); Web oWebsite = clientContext.Web; UserCustomActionCollection collUserCustomAction = oWebsite.UserCustomActions; UserCustomAction oUserCustomAction = collUserCustomAction.Add(); oUserCustomAction.Location = "Microsoft.SharePoint.StandardMenu"; oUserCustomAction.Group = "SiteActions"; oUserCustomAction.Sequence = 101; oUserCustomAction.Title = "Website User Custom Action"; oUserCustomAction.Description = "This description appears on the Site Actions menu."; oUserCustomAction.Url = urlWebsite + @"/_layouts/MyPage.aspx"; oUserCustomAction.Update(); clientContext.Load(oWebsite, webSite => webSite.UserCustomActions); clientContext.ExecuteQuery(); } } } Break Role Assignment Inheritance using System; using Microsoft.SharePoint.Client; using SP = Microsoft.SharePoint.Client; namespace Microsoft.SDK.SharePointServices.Samples { class BreakSecurityInheritance { static void Main() { string siteUrl = "http://MyServer/sites/MySiteCollection"; ClientContext oContext = new ClientContext(siteUrl); SP.List oList = oContext.Web.Lists.GetByTitle("Announcements"); oList.BreakRoleInheritance(true, false); oContext.ExecuteQuery(); } } } Breaking the security inheritance of a document and adding a user as reader using System; using Microsoft.SharePoint.Client; using SP = Microsoft.SharePoint.Client; namespace Microsoft.SDK.SharePointServices.Samples { class BreakSecurityInheritanceAddUser { static void Main() { string siteUrl = "http://MyServer/sites/MySiteCollection"; ClientContext clientContext = new ClientContext(siteUrl); SP.List oList = clientContext.Web.Lists.GetByTitle("MyList"); int itemId = 3; ListItem oListItem = oList.Items.GetById(itemId); oListItem.BreakRoleInheritance(false); User oUser = clientContext.Web.SiteUsers.GetByLoginName(@"DOMAIN\alias"); RoleDefinitionBindingCollection collRoleDefinitionBinding = new RoleDefinitionBindingCollection(clientContext); collRoleDefinitionBinding.Add(clientContext.Web.RoleDefinitions.GetByType(RoleType.Reader)); oListItem.RoleAssignments.Add(oUser, collRoleDefinitionBinding); clientContext.ExecuteQuery(); } } } Breaking the security inheritance of a document and changing the permissions of a user using System; using Microsoft.SharePoint.Client; using SP = Microsoft.SharePoint.Client; namespace Microsoft.SDK.SharePointServices.Samples { class BreakSecurityInheritanceChangeUser { static void Main() { string siteUrl = "http://MyServer/sites/MySiteCollection"; ClientContext clientContext = new ClientContext(siteUrl); SP.List oList = clientContext.Web.Lists.GetByTitle("MyList"); int itemId = 2; ListItem oListItem = oList.Items.GetById(itemId); oListItem.BreakRoleInheritance(true); User oUser = clientContext.Web.SiteUsers.GetByLoginName(@"DOMAIN\alias"); oListItem.RoleAssignments.GetByPrincipal(oUser).DeleteObject(); RoleDefinitionBindingCollection collRollDefinitionBinding = new RoleDefinitionBindingCollection(clientContext); collRollDefinitionBinding.Add(clientContext.Web.RoleDefinitions.GetByType(RoleType.Reader)); oListItem.RoleAssignments.Add(oUser, collRollDefinitionBinding); clientContext.ExecuteQuery(); } } }
All the question that scared me now i am trying to scare them .. so that they cant scare others :)
Friday, November 11, 2011
Managed Client Object Models in SharePoint Foundation 201
Subscribe to:
Post Comments (Atom)
Featured Posts
LM Studio is a desktop application designed for developing and experimenting with large language models (LLMs) directly on your computer.
LM Studio Overview LM Studio is a desktop application designed for developing and experimenting with large language models (LLMs...
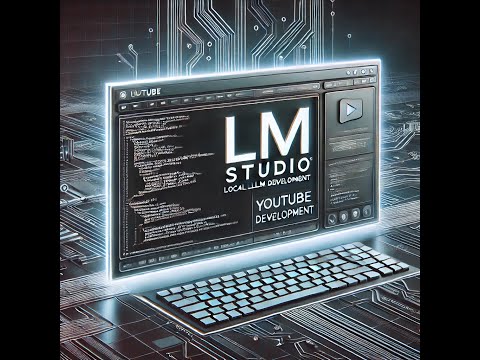
-
Configuration config = HBaseConfiguration.create(); Job job = new Job(config,"ExampleReadWrite"); job.setJarByClass(MyReadWriteJo...
-
All data is retrieved through a WitsmlServer instance which represents the WITSML server in the client program. There are three differe...
No comments:
Post a Comment
Thank you for Commenting Will reply soon ......